void zxfCOMPRESS_LONG(u32 t) {
u32 x = t, t1, t2, t3, t4,t5;
printBinary("x", (&x), 4);
x &= 0x92492492;
//x &= 0xff;
printBinary("x: x &= 0x92492492", (u8*)(&x), 4);
//printBinary("x: x &= 0xff", (u8*)(&x), 4);
t5 = (x << 2);
printBinary("t5 = (x << 2)", (u8*)(&t5), 4);
t1 = (x |t5 );
printBinary("t1: t1 =(x |t5 )", (u8*)(&t1), 4);
x = t1 & 0xc30c30c3;
printBinary("x: x = t1 & 0xc30c30c3;", (u8*)(&x), 4);
t5 = (x << 4);
printBinary("t5 = (x << 4)", (u8*)(&t5), 4);
t2 = (x | t5);
printBinary("t2: t2 = (x |t5);", (u8*)(&t2), 4);
x = t2 & 0xf00f00f0;
printBinary("x: x = t2 & f00f00f0;", (u8*)(&x), 4);
t5 = (x <<8);
printBinary("t5 = (x << 8)", (u8*)(&t5), 4);
t3 = (x | t5);
printBinary("t3: t3 = (x | t5);", (u8*)(&t3), 4);
x = t3 & 0xff0000ff;
printBinary("x: x = t3 & 0x00ff00ff;", (u8*)(&x), 4);
t5 = (x << 16);
printBinary("t5 = (x << 16)", (u8*)(&t5), 4);
t4 = (x | t5);
printBinary("t4: t4 = (x | t5);", (u8*)(&t4), 4);
x = t4 & 0xfff00000;
printBinary("x:x = t4 & 0xfff00000;", (u8*)(&x), 4);
t++;
}
void printBinary(unsigned char * str, u8 *a, int len) {
printf("%8s:\n", str);
int i, j, count = 0;
u8 t;
for (i = len-1; i >=0; i--) {
t = a[i];
for (j = 0; j < 8; j++) {
printf("%d,", t / 0x80);// 下标 ,取最高位
t = t * 2;
}
printf(" ");
}
printf("\n");
for (i = 0; i < len; i++) {
t = a[i];
printf("0x%02x : ", t);
for (j = 0; j < 8; j++) {
printf("[%02d]%d ", (len - 1 - i) * 8 + j, t / 0x80);// 下标 ,取最高位
t = t * 2;
}
printf("\n");
}
printf("\n");
}
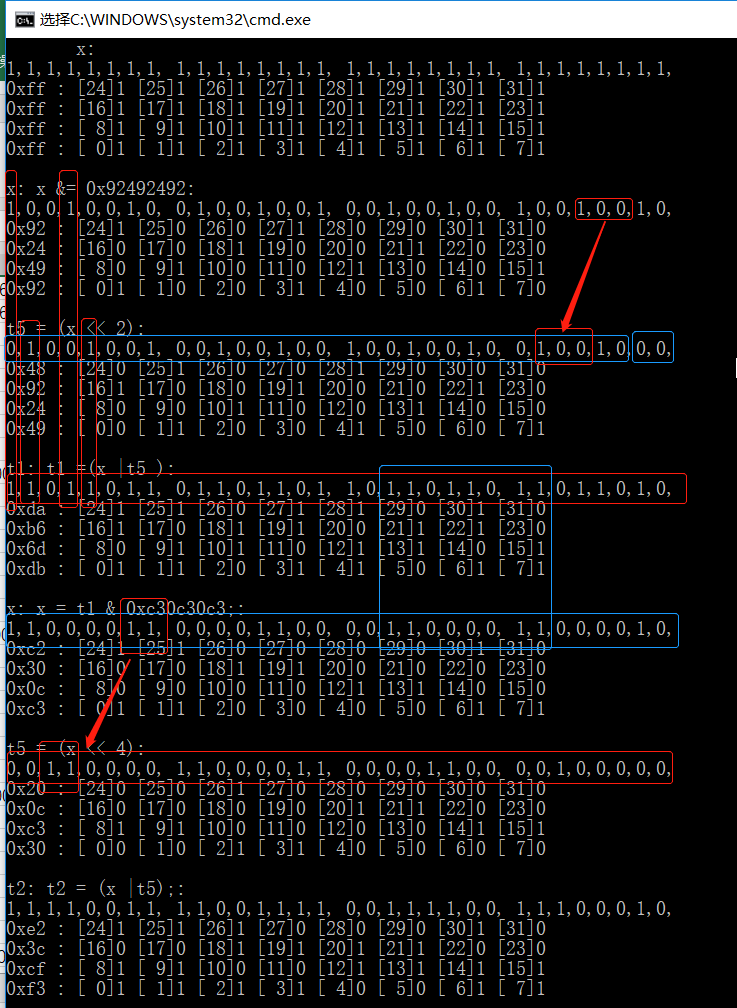
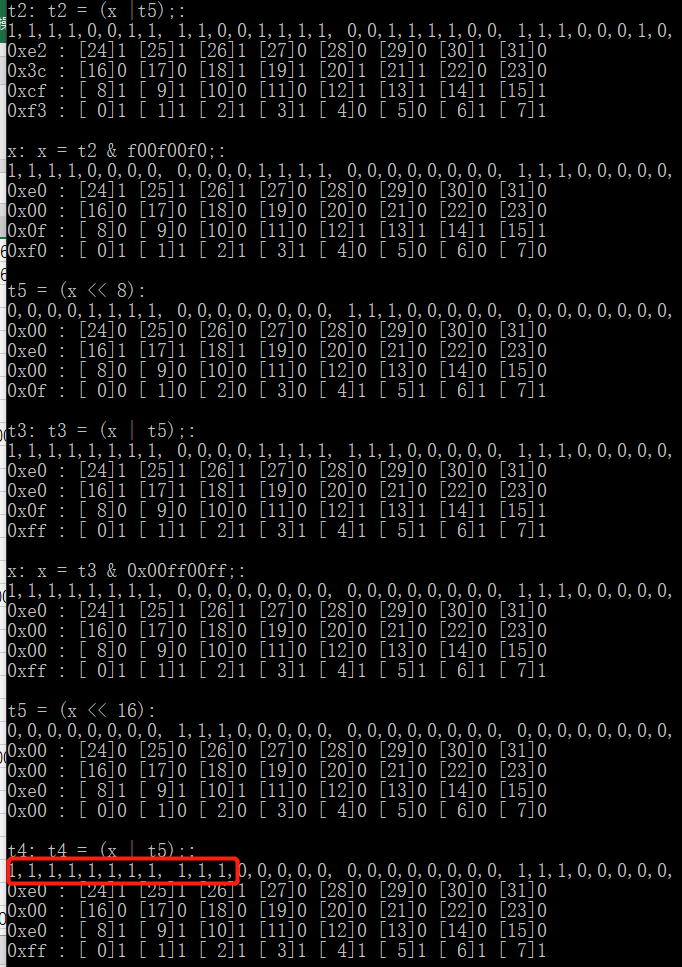
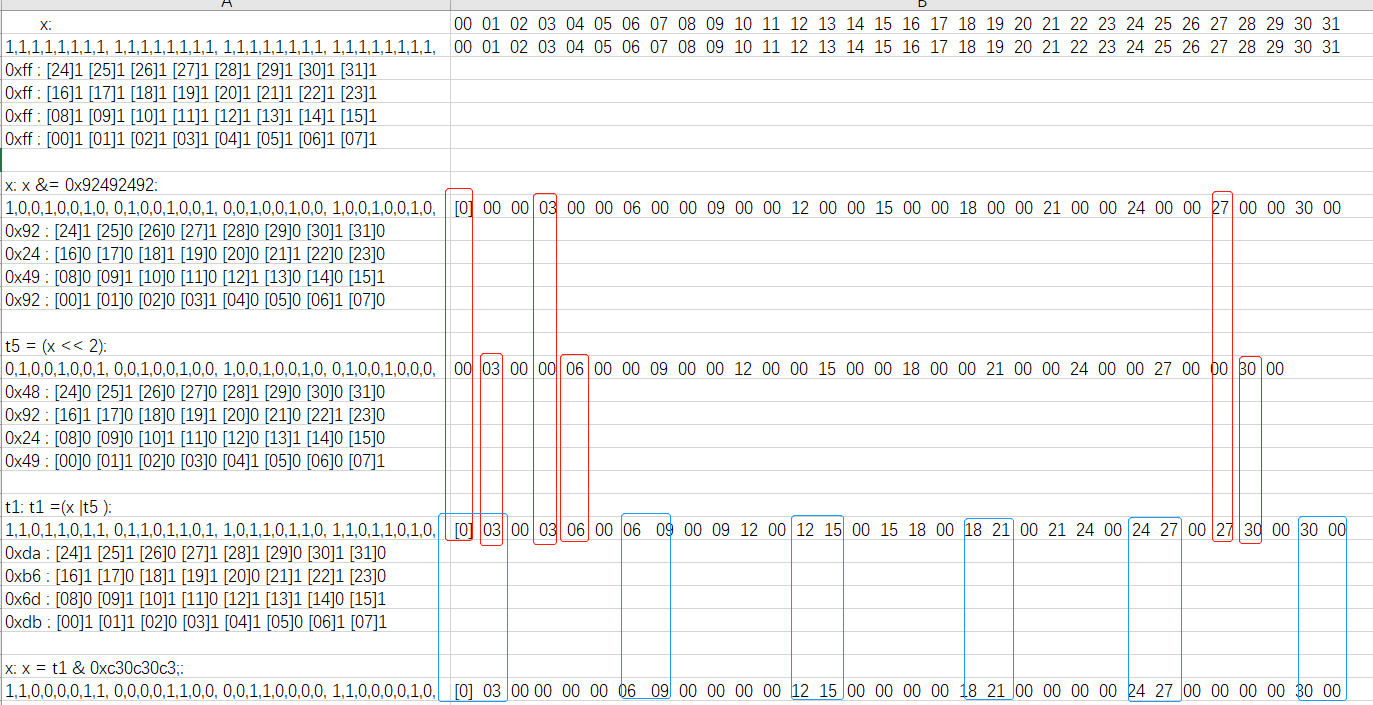
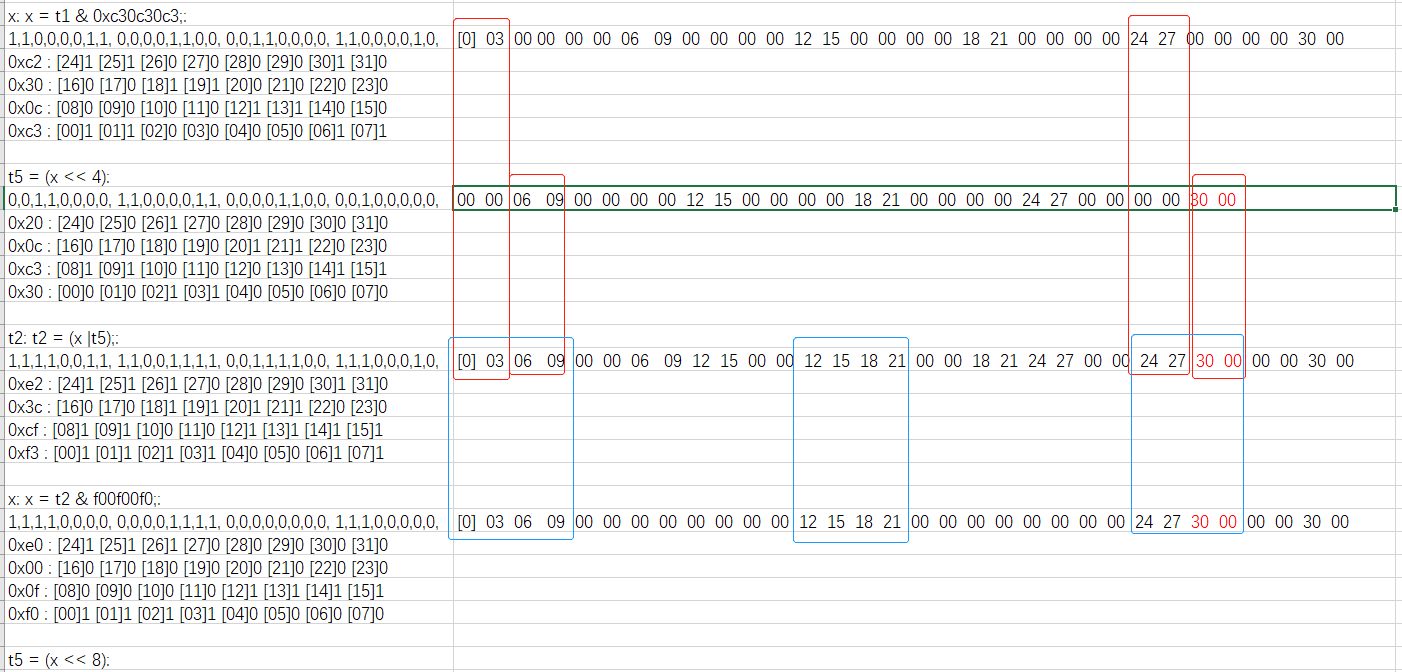
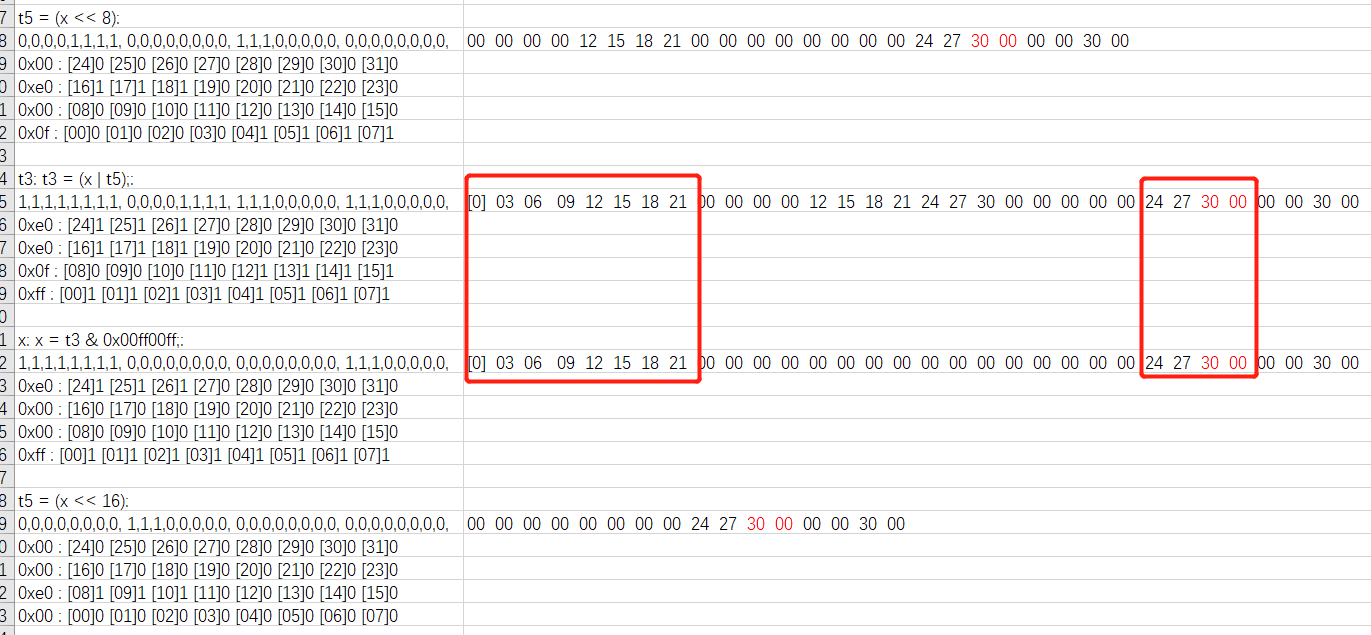
优化
#define puckU32ToThree_1(x){\ x &= 0x49249249;\ x = (x | (x >> 2)) & 0xc30c30c3;\ x = (x | (x >>4)) & 0x0f00f00f;\ x = (x | (x >> 8)) & 0xff0000ff;\ x = (x | (x >> 16)) & 0xfff;\ }
#define unpuckU32ToThree_1(x){\ x &= 0xfff;\ x = (x | (x << 16)) & 0xff0000ff;\ x = (x | (x << 8)) & 0x0f00f00f;\ x = (x | (x << 4)) & 0xc30c30c3;\ x = (x | (x << 2)) & 0x49249249;\ }
void packU96FormatToThreePacket1(u32 * out, u8 * in) {
u32 temp0[3] = { 0 };
u32 temp1[3] = { 0 };
u32 temp2[3] = { 0 };
temp0[0] = U32BIG(((u32*)in)[0]); temp0[1] = U32BIG(((u32*)in)[0]) >> 1; temp0[2] = U32BIG(((u32*)in)[0]) >> 2;
puckU32ToThree_1(temp0[0]);
puckU32ToThree_1(temp0[1]);
puckU32ToThree_1(temp0[2]);
temp1[0] = U32BIG(((u32*)in)[1]); temp1[1] = U32BIG(((u32*)in)[1]) >>1; temp1[2] = U32BIG(((u32*)in)[1]) >> 2;
puckU32ToThree_1(temp1[0]);
puckU32ToThree_1(temp1[1]);
puckU32ToThree_1(temp1[2]);
temp2[0] = U32BIG(((u32*)in)[2]); temp2[1] = U32BIG(((u32*)in)[2]) >> 1; temp2[2] = U32BIG(((u32*)in)[2]) >> 2;
puckU32ToThree_1(temp2[0]);
puckU32ToThree_1(temp2[1]);
puckU32ToThree_1(temp2[2]);
out[0] = (temp2[1]<<21) |(temp1[0]<<10) |temp0[2];
out[1] = (temp2[0] << 21) | (temp1[2] << 11) | temp0[1];
out[2] = (temp2[2] << 22) | (temp1[1] << 11) | temp0[0];
}
void unpackU96FormatToThreePacket(u8 * out, u32 * in) {
u32 temp0[3] = { 0 };
u32 temp1[3] = { 0 };
u32 temp2[3] = { 0 };
u32 t1_32, t2_64, t2_65;
u32 t[3] = { 0 };
temp0[0] = in[2] & 0x7ff;
temp0[1] = in[1] & 0x7ff;
temp0[2] = in[0] & 0x3ff;
temp1[0] = (in[0]>>10) & 0x7ff;
temp1[1] = (in[2] >>11 ) & 0x7ff;
temp1[2] = (in[1] >> 11) & 0x3ff;
temp2[0] = in[1] >> 21;
temp2[1] = in[0] >> 21;
temp2[2] = in[2] >> 22;
unpuckU32ToThree_1(temp0[0]);
unpuckU32ToThree_1(temp0[1]);
unpuckU32ToThree_1(temp0[2]);
t[0] = temp0[0] | temp0[1] << 1 | temp0[2] << 2;
unpuckU32ToThree_1(temp1[0]);
unpuckU32ToThree_1(temp1[1]);
unpuckU32ToThree_1(temp1[2]);
t[1] = temp1[0] | temp1[1] << 1 | temp1[2] << 2;
unpuckU32ToThree_1(temp2[0]);
unpuckU32ToThree_1(temp2[1]);
unpuckU32ToThree_1(temp2[2]);
t[2] = temp2[0] | temp2[1] << 1 | temp2[2] << 2;
memcpy(out, t, 12 * sizeof(unsigned char));
}

还没有评论,来说两句吧...